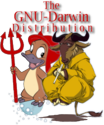
|
Once you have selected a graphics mode, you can draw things onto the display
via the 'screen' bitmap. All the Allegro graphics routines draw onto BITMAP
structures, which are areas of memory containing rectangular images, stored
as packed byte arrays (in 8 bit modes one byte per pixel, in 15 and 16 bit
modes sizeof(short) bytes per pixel, in 24 bit modes 3 bytes per pixel and
in 32 bit modes sizeof(long) bytes per pixel). You can create and manipulate
bitmaps in system RAM, or you can write to the special 'screen' bitmap which
represents the video memory in your graphics card.
For example, to draw a pixel onto the screen you would write:
putpixel(screen, x, y, color);
Or to implement a double-buffered system:
BITMAP *bmp = create_bitmap(320, 200); // make a bitmap in system RAM
clear_bitmap(bmp); // zero the memory bitmap
putpixel(bmp, x, y, color); // draw onto the memory bitmap
blit(bmp, screen, 0, 0, 0, 0, 320, 200); // copy it to the screen
See below for information on how to get direct access to the image memory in
a bitmap.
Allegro supports several different types of bitmaps:
- The screen bitmap, which represents the hardware video memory.
Ultimately you have to draw onto this in order for your image to be
visible.
- Memory bitmaps, which are located in system RAM and can be used to
store graphics or as temporary drawing spaces for double buffered
systems. These can be obtained by calling create_bitmap(), load_pcx(),
or by loading a grabber datafile.
- Sub-bitmaps. These share image memory with a parent bitmap (which
can be the screen, a memory bitmap, or another sub-bitmap), so drawing
onto them will also change their parent. They can be of any size and
located anywhere within the parent bitmap, and can have their own
clipping rectangles, so they are a useful way of dividing a bitmap into
several smaller units, eg. splitting a large virtual screen into
multiple sections (see examples/exscroll.c).
- Video memory bitmaps. These are created by the create_video_bitmap()
function, and are usually implemented as sub-bitmaps of the screen
object.
- System bitmaps. These are created by the create_system_bitmap()
function, and are a sort of halfway house between memory and video
bitmaps. They live in system memory, so you aren't limited by the
amount of video ram in your card, but they are stored in a
platform-specific format that may enable better hardware acceleration
than is possible with a normal memory bitmap (see the
GFX_HW_SYS_TO_VRAM_BLIT and GFX_HW_SYS_TO_VRAM_BLIT_MASKED flags in
gfx_capabilities). System bitmaps must be accessed in the same way as
video bitmaps, using the bank switch functions and bmp_write*() macros.
Not every platform implements this type of bitmap: if they aren't
available, create_system_bitmap() will function identically to
create_bitmap().
extern BITMAP *screen;
Global pointer to a bitmap, sized VIRTUAL_W x VIRTUAL_H. This is created
by set_gfx_mode(), and represents the hardware video memory. Only a part
of this bitmap will actually be visible, sized SCREEN_W x SCREEN_H.
Normally this is the top left corner of the larger virtual screen, so you
can ignore the extra invisible virtual size of the bitmap if you aren't
interested in hardware scrolling or page flipping. To move the visible
window to other parts of the screen bitmap, call scroll_screen().
Initially the clipping rectangle will be limited to the physical screen
size, so if you want to draw onto a larger virtual screen space outside
this rectangle, you will need to adjust the clipping.
BITMAP *create_bitmap(int width, int height);
Creates a memory bitmap sized width by height, and returns a pointer to
it. The bitmap will have clipping turned on, and the clipping rectangle
set to the full size of the bitmap. The image memory will not be cleared,
so it will probably contain garbage: you should clear the bitmap before
using it. This routine always uses the global pixel format, as specified
by calling set_color_depth().
BITMAP *create_bitmap_ex(int color_depth, int width, int height);
Creates a bitmap in a specific color depth (8, 15, 16, 24 or 32 bits per
pixel).
BITMAP *create_sub_bitmap(BITMAP *parent, int x, y, width, height);
Creates a sub-bitmap, ie. a bitmap sharing drawing memory with a
pre-existing bitmap, but possibly with a different size and clipping
settings. When creating a sub-bitmap of the mode-X screen, the x position
must be a multiple of four. The sub-bitmap width and height can extend
beyond the right and bottom edges of the parent (they will be clipped),
but the origin point must lie within the parent region.
BITMAP *create_video_bitmap(int width, int height);
Allocates a video memory bitmap of the specified size, returning a
pointer to it on success or NULL on failure (ie. if you have run out of
vram). This can be used to allocate offscreen video memory for storing
source graphics ready for a hardware accelerated blitting operation, or
to create multiple video memory pages which can then be displayed by
calling show_video_bitmap(). Video memory bitmaps are usually allocated
from the same space as the screen bitmap, so they may overlap with it: it
is not therefore a good idea to use the global screen at the same time as
any surfaces returned by this function.
BITMAP *create_system_bitmap(int width, int height);
Allocates a system memory bitmap of the specified size, returning a
pointer to it on success or NULL on failure.
void destroy_bitmap(BITMAP *bitmap);
Destroys a memory bitmap, sub-bitmap, video memory bitmap, or system
bitmap when you are finished with it.
void lock_bitmap(BITMAP *bitmap);
Under DOS, locks all the memory used by a bitmap. You don't normally need
to call this function unless you are doing very weird things in your
program.
int bitmap_color_depth(BITMAP *bmp);
Returns the color depth of the specified bitmap (8, 15, 16, 24, or 32).
int bitmap_mask_color(BITMAP *bmp);
Returns the mask color for the specified bitmap (the value which is
skipped when drawing sprites). For 256 color bitmaps this is zero, and
for truecolor bitmaps it is bright pink (maximum red and blue, zero
green).
int is_same_bitmap(BITMAP *bmp1, BITMAP *bmp2);
Returns TRUE if the two bitmaps describe the same drawing surface, ie.
the pointers are equal, one is a sub-bitmap of the other, or they are
both sub-bitmaps of a common parent.
int is_linear_bitmap(BITMAP *bmp);
Returns TRUE if bmp is a linear bitmap, ie. a memory bitmap, mode 13h
screen, or SVGA screen. Linear bitmaps can be used with the _putpixel(),
_getpixel(), bmp_write_line(), and bmp_read_line() functions.
int is_planar_bitmap(BITMAP *bmp);
Returns TRUE if bmp is a planar (mode-X or Xtended mode) screen bitmap.
int is_memory_bitmap(BITMAP *bmp);
Returns TRUE if bmp is a memory bitmap, ie. it was created by calling
create_bitmap() or loaded from a grabber datafile or image file. Memory
bitmaps can be accessed directly via the line pointers in the bitmap
structure, eg. bmp->line[y][x] = color.
int is_screen_bitmap(BITMAP *bmp);
Returns TRUE if bmp is the screen bitmap, or a sub-bitmap of the screen.
int is_video_bitmap(BITMAP *bmp);
Returns TRUE if bmp is the screen bitmap, a video memory bitmap, or a
sub-bitmap of either.
int is_system_bitmap(BITMAP *bmp);
Returns TRUE if bmp is a system bitmap object, or a sub-bitmap of one.
int is_sub_bitmap(BITMAP *bmp);
Returns TRUE if bmp is a sub-bitmap.
void acquire_bitmap(BITMAP *bmp);
Locks the specified video memory bitmap prior to drawing onto it. This
does not apply to memory bitmaps, and only affects some platforms
(Windows needs it, DOS does not). These calls are not strictly required,
because the drawing routines will automatically acquire the bitmap before
accessing it, but locking a DirectDraw surface is very slow, so you will
get much better performance if you acquire the screen just once at the
start of your main redraw function, and only release it when the drawing
is completely finished. Multiple acquire calls may be nested, and the
bitmap will only be truly released when the lock count returns to zero.
Be warned that DirectX programs activate a mutex lock whenever a surface
is locked, which prevents them from getting any input messages, so you
must be sure to release all your bitmaps before using any timer,
keyboard, or other non-graphics routines!
void release_bitmap(BITMAP *bmp);
Releases a bitmap that was previously locked by calling acquire_bitmap().
If the bitmap was locked multiple times, you must release it the same
number of times before it will truly be unlocked.
void acquire_screen();
Shortcut version of acquire_bitmap(screen);
void release_screen();
Shortcut version of release_bitmap(screen);
void set_clip(BITMAP *bitmap, int x1, int y1, int x2, int y2);
Each bitmap has an associated clipping rectangle, which is the area of
the image that it is ok to draw on. Nothing will be drawn to positions
outside this space. Pass the two opposite corners of the clipping
rectangle: these are inclusive, eg. set_clip(bitmap, 16, 16, 32, 32) will
allow drawing to (16, 16) and (32, 32), but not to (15, 15) and (33, 33).
If x1, y1, x2, and y2 are all zero, clipping will be turned off, which
may slightly speed up some drawing operations (usually a negligible
difference, although every little helps) but will result in your program
dying a horrible death if you try to draw beyond the edges of the bitmap.
|